Dev C%2b%2b Dictionary
Posted : admin On 17.12.2020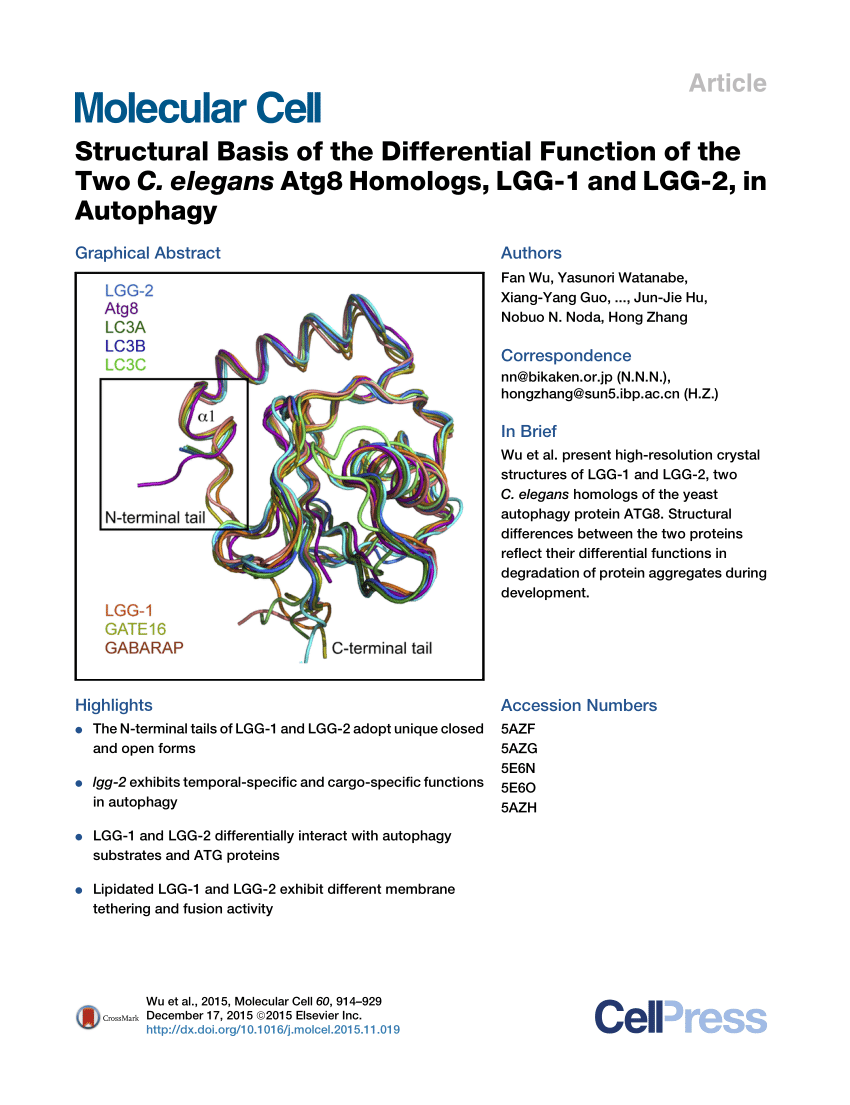
For that same reason, it is essential that all C programs have a main function. The word main is followed in the code by a pair of parentheses ( ). That is because it is a function declaration: In C, what differentiates a function declaration from other types of expressions are these parentheses that follow its name.
Language | ||||
Standard Library Headers | ||||
Freestanding and hosted implementations | ||||
Named requirements | ||||
Language support library | ||||
Concepts library(C++20) | ||||
Diagnostics library | ||||
Utilities library | ||||
Strings library | ||||
Containers library | ||||
Iterators library | ||||
Ranges library(C++20) | ||||
Algorithms library | ||||
Numerics library | ||||
Localizations library | ||||
Input/output library | ||||
Filesystem library(C++17) | ||||
Regular expressions library(C++11) | ||||
Atomic operations library(C++11) | ||||
Thread support library(C++11) | ||||
Technical Specifications |
C++98, C++03, C++11, C++14, C++17, C++20, C++23 | ||
Compiler support (11, 14, 17, 20) Basic concepts Feature test macros(C++20) Type support − traits(C++11) | Concepts library(C++20) Smart pointers and allocators basic_string array(C++11) − vector | Ranges library(C++20) Common math functions Stream-based I/O Filesystem library(C++17) Regular expressions library(C++11) Atomic operations library(C++11) atomic − atomic_flag Thread support library(C++11) |
Technical specifications Standard library extensions(library fundamentals TS) Standard library extensions v2(library fundamentals TS v2) propagate_const —ostream_joiner —randint Standard library extensions v3(library fundamentals TS v3) scope_exit —scope_fail —scope_success —unique_resource Concurrency library extensions(concurrency TS) | ||
External Links − Non-ANSI/ISO Libraries − Index − std Symbol Index |
Dev-C translation in Portuguese-English dictionary. Found 73 sentences matching phrase 'Dev-C'.Found in 20 ms. A software developer: a game dev; a web dev.
Stack Overflow for Teams is a private, secure spot for you and your coworkers to find and share information. Microsoft C/C lets you redefine a macro if the new definition is syntactically identical to the original definition. In other words, the two definitions can have different parameter names. This behavior differs from ANSI C, which requires that the two definitions be lexically identical.
- C++ Basics
- C++ Object Oriented
- C++ Advanced
- C++ Useful Resources
- Selected Reading
C/C++ arrays allow you to define variables that combine several data items of the same kind, but structure is another user defined data type which allows you to combine data items of different kinds.
Structures are used to represent a record, suppose you want to keep track of your books in a library. You might want to track the following attributes about each book −
- Title
- Author
- Subject
- Book ID
Defining a Structure
To define a structure, you must use the struct statement. The struct statement defines a new data type, with more than one member, for your program. The format of the struct statement is this −
The structure tag is optional and each member definition is a normal variable definition, such as int i; or float f; or any other valid variable definition. At the end of the structure's definition, before the final semicolon, you can specify one or more structure variables but it is optional. Here is the way you would declare the Book structure −
Accessing Structure Members
To access any member of a structure, we use the member access operator (.). The member access operator is coded as a period between the structure variable name and the structure member that we wish to access. You would use struct keyword to define variables of structure type. Following is the example to explain usage of structure −
When the above code is compiled and executed, it produces the following result −
Structures as Function Arguments
You can pass a structure as a function argument in very similar way as you pass any other variable or pointer. You would access structure variables in the similar way as you have accessed in the above example −
Dev C 2b 2b Dictionary Ap
When the above code is compiled and executed, it produces the following result −
Pointers to Structures
You can define pointers to structures in very similar way as you define pointer to any other variable as follows −
Now, you can store the address of a structure variable in the above defined pointer variable. To find the address of a structure variable, place the & operator before the structure's name as follows −
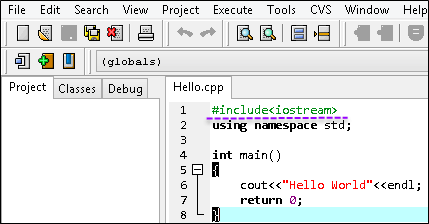
To access the members of a structure using a pointer to that structure, you must use the -> operator as follows −
Let us re-write above example using structure pointer, hope this will be easy for you to understand the concept −
When the above code is compiled and executed, it produces the following result −
The typedef Keyword
Dev C 2b 2b Dictionary Math
There is an easier way to define structs or you could 'alias' types you create. For example −
Now, you can use Books directly to define variables of Books type without using struct keyword. Following is the example −
You can use typedef keyword for non-structs as well as follows −
x, y and z are all pointers to long ints.